Mastering Debugging in Symfony
Coding (Symfony)
Discover effective techniques and tools to squash bugs and optimize performance

What if I tell you I spent the last 40 hours of my work week debugging an issue on my Symfony application?
Sounds like a nightmare? well, pretty close.
In this post, I’ll show you what I did to fix the issue and how I make my program more reliable than ever.
Effective debugging not only will save me time from now on but also improves the overall quality of my (and your) code.
Debugging is not merely about fixing bugs; it is a powerful tool that empowers us to identify and resolve issues rapidly.
Imagine encountering a bug that halts your progress or causes unexpected behavior.
With efficient debugging skills, you can pinpoint the root cause, apply effective solutions, and keep your development on track.
By actively debugging, you can prevent future issues, improve code readability, and foster a more maintainable and scalable Symfony application.
In this blog post, we will explore the importance of debugging in Symfony applications and discover tips and techniques to enhance your development process.
Understanding Symfony Debugging Tools
Symfony, being a robust PHP framework, offers a suite of powerful built-in debugging components that can greatly assist developers in troubleshooting and optimizing their applications.
These components provide valuable insights into the application’s execution flow, performance, and potential issues.
Let’s explore some of the key debugging components that Symfony provides.
To make the most of Symfony’s debugging capabilities, it’s essential to set up and configure your debugging environment properly.
This involves enabling the debug mode, which provides detailed error messages and stack traces, and ensuring that the necessary debugging tools are installed and configured.
By having a well-configured debugging environment, you can efficiently identify and resolve issues during the development and testing stages.
One of the standout features of Symfony is its Profiler and Web Debug Toolbar, which offer extensive insights into the application’s performance, database queries, executed code, and more.
The Profiler provides a detailed timeline of the application’s request-response cycle, allowing you to analyze the execution flow and identify any potential bottlenecks or inefficiencies.
The Web Debug Toolbar, on the other hand, offers a convenient way to access essential debugging information directly within the browser, such as the number of database queries executed, memory usage, and more.
By leveraging the Symfony Profiler and Web Debug Toolbar, you can gain a deeper understanding of your application’s behavior, identify performance optimizations, and quickly diagnose and fix potential issues.
Common Debugging Techniques
Effective debugging is a vital skill for every Symfony developer.
It allows you to identify and fix issues quickly, resulting in improved code quality and faster development cycles.
Utilizing error logs and exception handling:
Symfony provides a robust logging mechanism that allows you to capture and store important information about errors and exceptions.
By configuring your application to log errors, you can review the logs to gain insights into what went wrong and where.
For example, you can use the following code snippet to log an error message:
try { // Your code here } catch (\Exception $e) { $logger->error(‘An error occurred: ‘ . $e->getMessage()); }
Debugging with var_dump and print_r
When troubleshooting specific variables or data structures, var_dump
(read the official docs) and print_r
functions can come in handy.
These functions allow you to inspect and print the contents of variables, arrays, or objects directly to the browser or console.
$data = [‘apple’, ‘banana’, ‘orange’]; var_dump($data);
Using Symfony Console component for command-line debugging
Symfony’s Console component provides a powerful command-line interface that can aid in debugging.
You can create custom commands to perform specific debugging tasks or interact with your application.
This allows you to execute debugging routines directly from the command line, making it easier to test and troubleshoot specific features.
Exploring the Xdebug extension for advanced debugging capabilities
Xdebug is a popular extension for PHP that provides advanced debugging features.
It integrates seamlessly with Symfony and offers functionalities like breakpoints, step debugging, variable inspection, and stack trace.
Installing and configuring Xdebug can greatly enhance your debugging capabilities, allowing you to dive deep into the code and pinpoint issues efficiently.
Advanced Debugging Techniques
As a Symfony developer, it’s crucial to have a repertoire of advanced debugging techniques in your toolkit.
These techniques go beyond basic troubleshooting and empower you to optimize performance, debug API requests, trace database queries, and monitor application logs.
Let’s explore these advanced debugging techniques:
Profiling Symfony applications for performance optimization
Profiling helps identify performance bottlenecks in your Symfony application.
By leveraging the profiler, you can pinpoint areas that require optimization and make informed decisions to improve your application’s performance.
// Enable profiling $kernel = new AppKernel(‘dev’, true);
Debugging API requests and responses:
When working with Symfony as an API backend, debugging requests and responses becomes essential.
By logging and inspecting API payloads, headers, and status codes, you can troubleshoot issues related to data transmission and integration.
Here’s an example of logging API requests using the Monolog library:
// Log API requests $logger->info(‘API Request’, [‘request’ => $request->toArray()]);
Tracing and debugging database queries
Efficiently debugging database queries is crucial for optimizing database performance.
Symfony’s Doctrine ORM provides powerful debugging tools that allow you to log and inspect SQL queries, query execution times, and result sets.
By tracing and analyzing database queries, you can identify and resolve inefficiencies.
Use the following code snippet to enable query logging:
// Enable query logging $entityManager ->getConnection() ->getConfiguration() ->setSQLLogger(new \Doctrine\DBAL\Logging\DebugStack());
Monitoring and analyzing application logs for bug detection
Application logs play a crucial role in identifying and resolving bugs.
By monitoring and analyzing logs, you can uncover hidden issues, exceptions, and error messages.
Symfony’s logging components, like Monolog, provide flexible log handling capabilities, allowing you to store logs in files, and databases, or even send them to external services for real-time monitoring.
Here’s an example of logging an error:
// Log an error $logger->error(‘An error occurred’, [‘exception’ => $e]);
By mastering these advanced debugging techniques, you can enhance your Symfony development workflow, optimize performance, and streamline bug detection and resolution.
Debugging in Production
Debugging a Symfony application in a production environment requires a different approach compared to a development or staging environment.
While you can’t rely on traditional debugging techniques, there are still effective ways to troubleshoot issues and ensure smooth operation.
Let’s explore some techniques for debugging live Symfony applications, along with tools and mechanisms to assist in the process.
Techniques for debugging live Symfony applications
Debugging in a production environment requires caution and minimal impact on the application’s performance.
Instead of modifying code directly, we can utilize techniques that provide insights into the application’s behavior without disrupting its functionality.
The first technique is logging (same as we saw above).
// Log important events and messages $this->logger->info(‘Processing request…’, [‘request’ => $request]);
By strategically placing logging statements throughout your codebase, you can gather valuable information about the application’s execution flow, variable values, and potential errors.
These logs can later be analyzed to identify and address any issues.
Log analysis and error-tracking tools
To streamline the debugging process, consider using log analysis and error-tracking tools specifically designed for production environments.
Tools like Sentry, New Relic, or Rollbar offer comprehensive error monitoring and reporting capabilities.
They collect and aggregate logs, exceptions, and errors, providing you with a centralized dashboard to investigate issues.
// Configure Symfony to send logs to an external service monolog: handlers: main: type: fingers_crossed action_level: error handler: nested nested: type: service id: my_custom_log_handler
Integrating such tools into your Symfony application allows you to proactively monitor and receive alerts for critical errors, ensuring that potential issues are swiftly addressed.
Implementing error reporting and handling mechanisms
To handle errors and exceptions gracefully in a production environment, it’s essential to implement error reporting and handling mechanisms.
Symfony provides robust error-handling capabilities through custom exception handlers.
// Custom exception handler class CustomExceptionHandler implements ExceptionHandlerInterface { public function handle(ExceptionEvent $event): void { // Handle and format the exception response } }
By implementing a custom exception handler, you can control how errors are displayed to users, log detailed error information, and provide appropriate responses.
Remember, debugging in a production environment requires extra caution to minimize disruptions and ensure a seamless experience for your users.
Leveraging logging, utilizing log analysis tools, and implementing error reporting mechanisms will help you identify and address issues efficiently.
Conclusion
Debugging is an essential aspect of PHP development, particularly when working with Symfony applications.
By investing time in effective debugging techniques, you can enhance the reliability and performance of your code.
Debugging enables you to gain insights into your application’s behavior, identify and fix bugs, and optimize its functionality.
It empowers you to create more reliable and robust PHP applications, leading to improved code quality and user experience.
I regularly share valuable insights and tips.
Join my email list to stay up to date with the latest blog posts, where I explore various topics related to PHP and web development. By subscribing, you can gain practical knowledge and take your Symfony development skills to new heights.
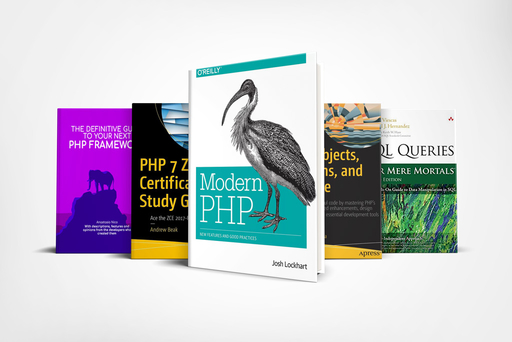