Visibility and Static keyword in PHP
Coding (Php 7.x)
Protect your data by leveraging the OOP concept of visibility

Follow the series ...
This blog post is the fourth part of "The complete guide to Object-Oriented Programming: Go from procedural programming to OOP expert in PHP"
If you did not read the other parts yet
You can check the other blog posts following the links below
Introduction to Object-Oriented Programming,
Inheritance and Interfaces in PHP,
More Interfaces and Polymorphism,
Visibility and Static keyword,
Constructor and Magic methods and
Abstract Classes and Extra bits
Introduction to the Visibility concept
Understanding visibility and scope is pretty straightforward,
but it can lead to some confusion if the background is not strong enough.
I do not know why but as always programmers like to define fancy words to describe simple concepts.
You have already seen some example with polymorphism, inheritance and now you are about to discover another word:
Encapsulation.
This elaborate word means nothing other than gathering data together in a way that the rest of the code does not affect it.
In the following example, you will learn about the concept of scope.
Given two variable with the same name called $schoolsCount, one counts all the
school in our city and the other only counts the number of schools in the main street you will see that the result will be different.
This happens because the second variable has been encapsulated within the function, thus allocated in a different scope.
We can use global scope by using the superglobal variable GLOBALS['schoolCount']
but, this is not a good practice and it will make the code very messy very soon.
$schoolsCount = 7; function getSchoolsCountInMainStreet () { $schoolsCount = 4; return var_dump($schoolsCount); } var_dump($schoolsCount); // This will output the total amount
In Object Oriented Programming with PHP, you have three different
levels of visibility.
They are:
- Public
- Protected
- Private
Public
Public is the first and least restricted level,
Properties and methods with this level can be accessed, viewed and updated from everywhere in the code, within a class, from a subclass or even outside the class.
The visibility has been featured after PHP4 and for retro compatibility
declare it is not mandatory, actually if you do not declare the visibility
on an element, the code will consider it public.
So,
consider that all the examples you have seen so far in this article have been publicly declared.
Sources say that this is not going to stay any longer, the web application deployed in PHP4 and still online are very few.
Which means you need to get the habit of declaring visibility even when methods and attributes are public for future compatibility reason
Protected
Protected is the second level.
And it is very easy to understand so I will not dwell much on it.
When declaring a property protected you are saying that this variable is available everywhere inside the class and in any class that extends it.
(see inheritance and interfaces for more about this topic)
Private
The third level is the more limited,
private properties and methods can be accessed only from within the class they are declared.
No subclass, no elsewhere.
This type of visibility is used for very sensitive information like when you are working with passwords or dealing with payment data.
PHP syntax orders us to define the visibility before writing the element.
Let’s rewrite a previous example:
class Building { public $inCostruction = false; protected $isOpen = true; private $safePassword = “qwerty” public function closeBuilding() { return $this->isOpen = false; } protected function openBuilding() { return $this->isOpen = true; } } class School extends Building { } class Office extends Building { $isOpen = false; }
Currently $inCostruction variable is accessible everywhere in the program,
$isOpen is visible in the Building class in the child classes whereas $safePassword is only visible in the Building class,
No School nor Office can access and update the $safePassword variable.
This is a very basic feature of PHP but must be clearly understood.
When getting the scope wrong on a variable the majority of the errors that will occur for visibility reasons are fatal errors
if there is no error shown it will be very arduous for you to find the problem at all.
Static
Here it is a new PHP keyword for you to learn.
They are never enough,
isn’t it?
The static keyword is used to change the accessibility of properties and
methods.
By declaring an element of a class (either properties or methods) as static you are saying to the code that the element will be available to all program without the necessity to instantiate the class.
Since there is no class instanciated the keyword $this is not available inside these methods.
It is replaced by the self:: keyword instead.
Confused?
let’s make thing a little bit more clear.
Why use static in the first place?
To do that we need to create copies of the Building class and all the actions we perform are going to change only the object instantiated and never the class itself.
This means that we can have dozens, hundreds or even thousands of Offices in our imaginary city and we can edit each of them without affecting the others.
$officeInMainStreet = new Office() $officeInPiccadillyBoulevard= new Office()
These two objects are two different instances of the same class,
They were built using the same blueprint but they are not the same office
You can add a window or repaint the wall on one of them and there will be no problem because the other object will never know.
Well, when using static things are different.
class Building { public static $planningPermission = ‘accepted’; } echo Building::$planningPermission; // this command will echo “accepted”
In the example above we are not trying to access an abject anymore but we are accessing the property directly,
So,
you are seeing echoed the actual static parameter of the actual class.
If you were to create a method that will end up editing the parameter it will be the parameter of the class that will be changed not the one of some instance create afterward.
The same worth for static methods:
class Building { public static $planningPermission = ‘accepted’; public static function setDeniedPermission() { self::$planningPermission = ‘denied’; return self::$planningPermission; } } echo Building::setDeniedPermission(); // this command will echo “denied”
This notion makes the static keyword very powerful but at the same time is not seen good from many developers, that consider its and the use of the global scope, in general, a bad practice.
Conclusion of the fourth part
This was a quick post that indicates you the easy concepts of visibility and use of the static keyword on PHP.
Even though these elements only require a basic level of knowledge and are applicable straight away,
nobody can deny the importance of their use,
especially, if properly applied.
In many ways,
choose a protected or private level of visibility helps the web developer define what is shareable and what it is better that stays within friendly walls.
At the same time,
you have learned that you can now declare classes without the necessity of instantiating them.
The static keyword is another powerful but dangerous feature of the language.
It is an elementary error to learn some new keywords and start to use them everywhere in your scripts.
but be careful,
a misuse of it, especially at the beginning, can lead at a complete rewrite of already finished features (it happened to me, learn from my mistakes).
In the next episode,
we will focus on magic methods
I have prepared a complete list with a detailed description and code examples.
For this reason,
Stay tuned and subscribe using the form down here to get notified when it will be published.
Now it is your turn:
Are you going to update your methods and properties applying protected and private keywords or do you prefer spread static keywords everywhere?
let me know in the comment box below!
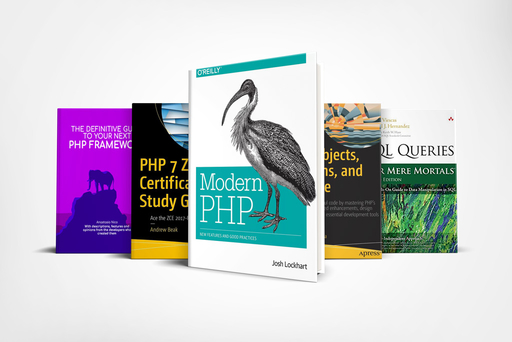