The Art of Code Design: Demystifying the Liskov Substitution Principle
Coding (Software architecture)
Learn how to enhance the flexibility and maintainability of your code with the Liskov substitution principle. Discover best practices and real-world examples for writing robust and extensible software
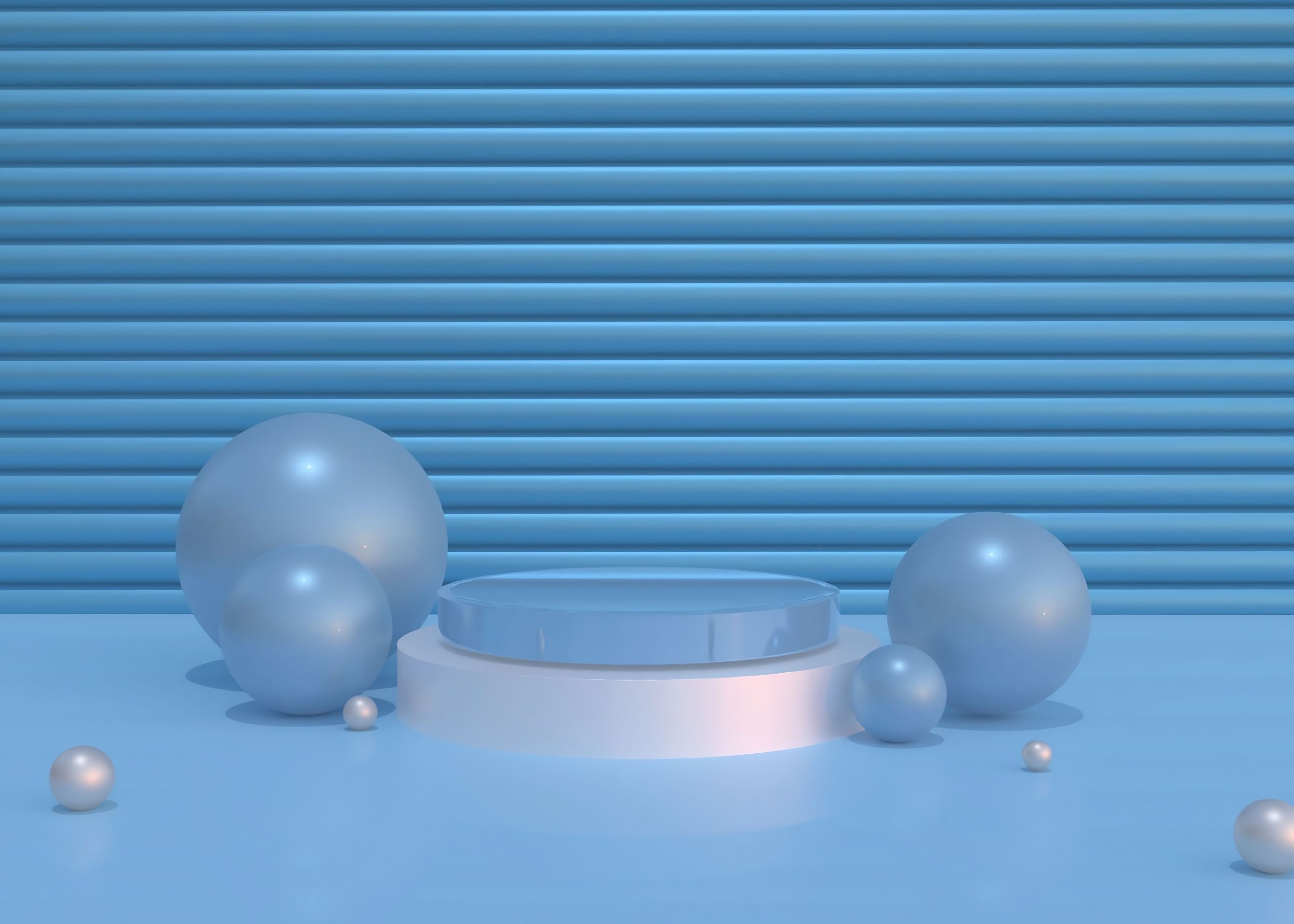
Imagine you're a skilled architect tasked with designing a magnificent skyscraper.
You meticulously plan every aspect, from the foundation to the intricate details of the facade.
But as construction begins, you realize that different teams are working on different sections of the building without adhering to a common set of guidelines.
The result?
Incompatible materials, inefficient workflows, and a structure that lacks cohesion.
In the world of software development, a similar scenario can unfold when the Liskov substitution principle is overlooked.
This principle emphasizes that objects of a superclass should be replaceable with objects of their subclasses without affecting the correctness or behavior of the program.
It's like ensuring that each piece of your skyscraper's puzzle fits perfectly, seamlessly blending together to create a cohesive and harmonious structure.
By following the Liskov substitution principle, you ensure that your code is built on a solid foundation, allowing for effortless extensibility and maintainability.
It promotes modularity and flexibility, enabling you to swap out components or add new features without causing unexpected side effects.
So, just as an architect strives for a structurally sound and visually stunning skyscraper, as a developer, you too can create elegant and reliable software by embracing the Liskov substitution principle.
Let's explore this principle further and discover how it can elevate the quality of your code.
Understanding the SOLID Principles
Let's talk SOLID principles! They are the guiding stars of software design, aiming for high-quality, flexible, and maintainable code.
These principles provide guidelines for creating robust software systems.
Here's a quick overview:
-
SRP (Single Responsibility Principle): Each class has one job, making code manageable and bug-resistant.
-
OCP (Open-Closed Principle): Code is open for extension but closed for modification, enabling new features without rewriting existing code.
-
LSP (Liskov Substitution Principle): Objects of a superclass can be replaced by subclasses seamlessly, promoting polymorphism.
-
ISP (Interface Segregation Principle): Keep interfaces focused and relevant to avoid unnecessary dependencies and ensure cleaner code.
-
DIP (Dependency Inversion Principle): Depend on abstractions instead of concrete implementations for loose coupling and improved testability.
I have already written about the Single Responsibility Principle and the Open-Closed Principle in the previous articles of this series.
in this post we are going to delve into the Liskov Substitution Principle.
Anyway, all these principles empower us to create clean, maintainable, and extensible code
The Liskov Substitution Principle (LSP)
Let's dive into the Liskov Substitution Principle (LSP), as said above this is one of the essential principles in the SOLID framework.
LSP focuses on the relationship between subclasses and their superclasses, ensuring that subtypes can be seamlessly substituted for their base types without impacting the correctness of the program.
At its core, LSP emphasizes the idea that objects of a superclass should be replaceable with objects of their subclasses without causing any unexpected behavior or breaking the program's logic.
In other words, if a piece of code is written to work with a certain type, it should continue to work correctly when using any subtype of that type.
By adhering to LSP, we achieve enhanced modularity and code reusability.
Subtypes that conform to LSP can be used interchangeably with their base types, allowing for flexible and extensible code.
This promotes modularity as each subclass can have its own specific behavior while still maintaining compatibility with the superclass.
Furthermore, LSP encourages better code organization and promotes the reuse of existing code.
When new subtypes can be seamlessly integrated into existing code without introducing bugs or requiring modifications, it becomes easier to leverage and extend the functionality of the codebase.
In our upcoming examples and discussions, we will explore practical scenarios where applying LSP leads to more robust and maintainable code.
By understanding and embracing LSP, you'll be equipped with the knowledge to design software systems that are flexible, scalable, and easy to extend.
Key Concepts of LSP
To fully grasp the Liskov Substitution Principle (LSP), it's crucial to understand the key concepts that underpin its implementation.
Admitelly, It took me quite a few years to fully understand this concept, I hope this will help you be quicker.
Let's explore two fundamental concepts: behavioral subtyping and contract compliance.
Behavioral subtyping lies at the heart of LSP.
It emphasizes that a subclass should exhibit the same behavior as its superclass, or in other words, it should honor the same set of obligations and fulfill the same expectations.
This concept ensures that a subtype can be used as a substitute for its base type without compromising the correctness of the program.
Contract compliance is closely tied to behavioral subtyping.
It refers to the idea that a subclass must adhere to the contract defined by its superclass.
The contract encompasses the methods, properties, and behavior that the superclass expects from its subclasses.
By complying with the contract, the subclass guarantees substitutability, allowing it to seamlessly replace instances of the superclass without causing any unexpected issues.
Let's illustrate these concepts with an example!
Suppose we have a class hierarchy representing different shapes: a base class called `Shape` and two subclasses, `Rectangle` and `Circle`. The `Shape` class defines a method called `calculateArea()`, which calculates and returns the area of the shape.
For behavioral subtyping, both the `Rectangle` and `Circle` classes should provide an implementation of the `calculateArea()` method.
Each subclass will compute the area based on its specific attributes (e.g., length and width for rectangles, radius for circles) but ensure that the method's purpose remains consistent across the hierarchy.
Regarding contract compliance, the `Rectangle` and `Circle` classes must fulfill the obligations set by the `Shape` class.
They should offer the same set of public methods, follow to any specified input/output behavior, and maintain the general semantics defined by the `Shape` contract.
This allows instances of `Rectangle` and `Circle` to be used interchangeably with `Shape` objects, enabling polymorphism and code reuse.
By grasping these key concepts of behavioral subtyping and contract compliance, you can effectively apply LSP in your software design.
Ensuring that subclasses exhibit the expected behavior and comply with the contracts set by their superclasses fosters code extensibility, maintainability, and robustness.
Real-world Examples of LSP Violations
While understanding the Liskov Substitution Principle (LSP) is essential for writing robust and maintainable code, it's equally important to recognize the potential consequences of violating this principle.
Let's explore some real-world examples where LSP violations led to design issues and code problems.
Consider a scenario where we have a class hierarchy representing shapes, with a base class called `Shape` and two subclasses, `Rectangle` and `Circle`.
I used this example in the previous blog post and you guys seem to really like so here they are again.
Each shape class has a method called `calculateArea()` to compute its area.
Now, suppose we introduce a new subclass called `Triangle`.
However, instead of implementing the `calculateArea()` method, we decide to override it with a completely different behavior, such as computing the perimeter of the triangle.
This violation of LSP introduces a design issue.
Clients relying on the `Shape` contract expect all subclasses to provide an implementation of `calculateArea()`.
By violating this expectation, we break the substitutability principle.
Code that interacts with shapes through the `Shape` type may rely on the presence and behavior of `calculateArea()`, assuming it computes the area.
Consequently, using an instance of `Triangle` as a substitute for a `Shape` object could lead to unexpected errors or incorrect calculations.
Let's take a closer look at the code to illustrate this violation:
The following are examples in PHP, if you need to brush up the sintax here is a article about the basics of PHP.
class Shape { // ... public function calculateArea() { // Calculate and return the area } // ... } class Triangle extends Shape { // ... public function calculateArea() { // Calculate and return the perimeter (violating LSP) } // ... }
In this example, the `Triangle` class violates the LSP by providing a different behavior for the `calculateArea()` method.
This can result in code that misuses or misunderstands the expected behavior, leading to errors and maintenance challenges.
By analyzing such violations, we can understand the potential impact on code maintainability.
When subclasses deviate from the expected behavior defined by their superclasses, it becomes difficult to reason about and work with instances of different subclasses interchangeably.
Code that relies on the `Shape` contract may assume specific behavior, leading to bugs and incorrect results when interacting with violating subclasses.
Avoiding LSP violations is crucial for maintaining a flexible and robust codebase.
Ensuring that subclasses observe to the expected behavior and fulfill the contracts defined by their superclasses promotes code extensibility, facilitates code reuse, and enhances overall software quality.
Benefits and Implications of LSP
The Liskov Substitution Principle (LSP) brings numerous benefits to software development when followed diligently.
Let's delve into the implications and advantages of adhering to LSP, highlighting how it contributes to improved code extensibility, robustness, and overall code quality.
By designing our codebase in accordance with LSP, we foster greater code extensibility.
Subclasses that conform to the behavior and contracts established by their superclasses can seamlessly integrate into existing code without introducing unexpected errors or breaking existing functionality.
They way I design the classes on my codebase is by writing UML scheme with the relationship of the classes beforehand.
This allows for the introduction of new features or variations of existing classes without the need for extensive modifications in other parts of the codebase.
Moreover, adhering to LSP enhances code robustness.
When we rely on behavioral subtyping, where subclasses can be treated as their base types, we establish a strong foundation for code stability.
This enables us to write code that can work with different implementations of the same base class, providing a consistent and reliable behavior across the application.
Following LSP also has implications for code quality, testability, and scalability.
By adhering to LSP, we encourage the development of code that is easier to test and reason about.
Since subclasses can be treated as their base types, it becomes simpler to write test cases that cover various scenarios without the need for specialized tests for each subclass.
This leads to improved test coverage and ensures that our code behaves as expected in different situations.
Additionally, LSP plays a vital role in facilitating code scalability.
As the codebase grows, adhering to LSP allows for the seamless addition of new classes or variations without causing disruptions to the existing code.
This promotes code modularity, making it easier to understand and maintain, even as the system becomes more complex.
Let's take a look at how observance to LSP can be demonstrated in the context of our shapes example:
class Shape { // Common shape methods and properties } class Rectangle extends Shape { // Rectangle-specific implementation } class Circle extends Shape { // Circle-specific implementation }
In this example, both the `Rectangle` and `Circle` classes conform to the behavior and contracts defined by their base class, `Shape`.
This adherence to LSP ensures that these subclasses can be used interchangeably wherever a `Shape` instance is expected, without compromising the correctness or behavior of the program.
By embracing the principles of LSP, we lay the foundation for building maintainable, extensible, and robust code.
The benefits of following the LSP include improved code extensibility, enhanced code robustness, and better testability. Furthermore, adhering to LSP promotes code scalability, allowing our systems to grow and evolve with ease.
Conclusion
We have explored the significance of the SOLID principles, with a particular focus on the Liskov Substitution Principle (LSP).
Let's recap the key points we have discussed throughout this blog post.
The SOLID principles serve as a set of guidelines for software design and development, promoting code quality, extensibility, and ease of maintenance.
The Liskov substitution principle, as one of the SOLID principles, emphasizes the importance of designing code in a way that allows subclasses to be substitutable for their base types without affecting the correctness of the program.
By adhering to LSP, developers can experience several benefits.
Improved code extensibility allows for the seamless integration of new features and variations without disrupting existing functionality.
Code robustness is enhanced as behavioral subtyping ensures consistent and reliable behavior across the application.
Additionally, adhering to LSP improves code quality, testability, and scalability.
As you embark on your software development projects, I encourage you to apply the Liskov substitution principle and following to the SOLID principles.
By doing so, you can achieve better code quality, maintainability, and scalability, leading to more robust and flexible software systems.
Stay tuned for upcoming blog posts in the SOLID principles series (3 more to come).
Each post will provide valuable insights and practical examples to further enhance your understanding and application of these principles.
Don't forget to subscribe to the newsletter to stay updated on the latest articles, resources, and tips related to web development and the SOLID principles.
By subscribing, you'll receive exclusive content and free reviews of the best books on web development that have helped me along my journey as a software engineer.
Thank you for joining me on this exploration of the Liskov substitution principle and its role in software design.
Together, let's build robust, scalable, and maintainable code that stands the test of time.
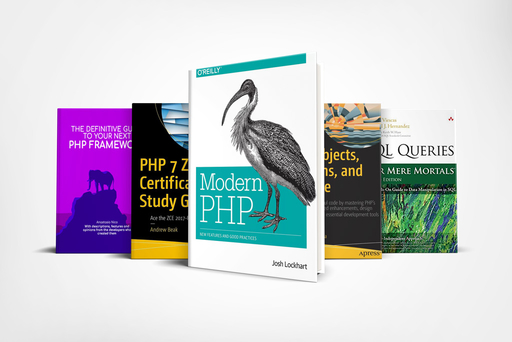